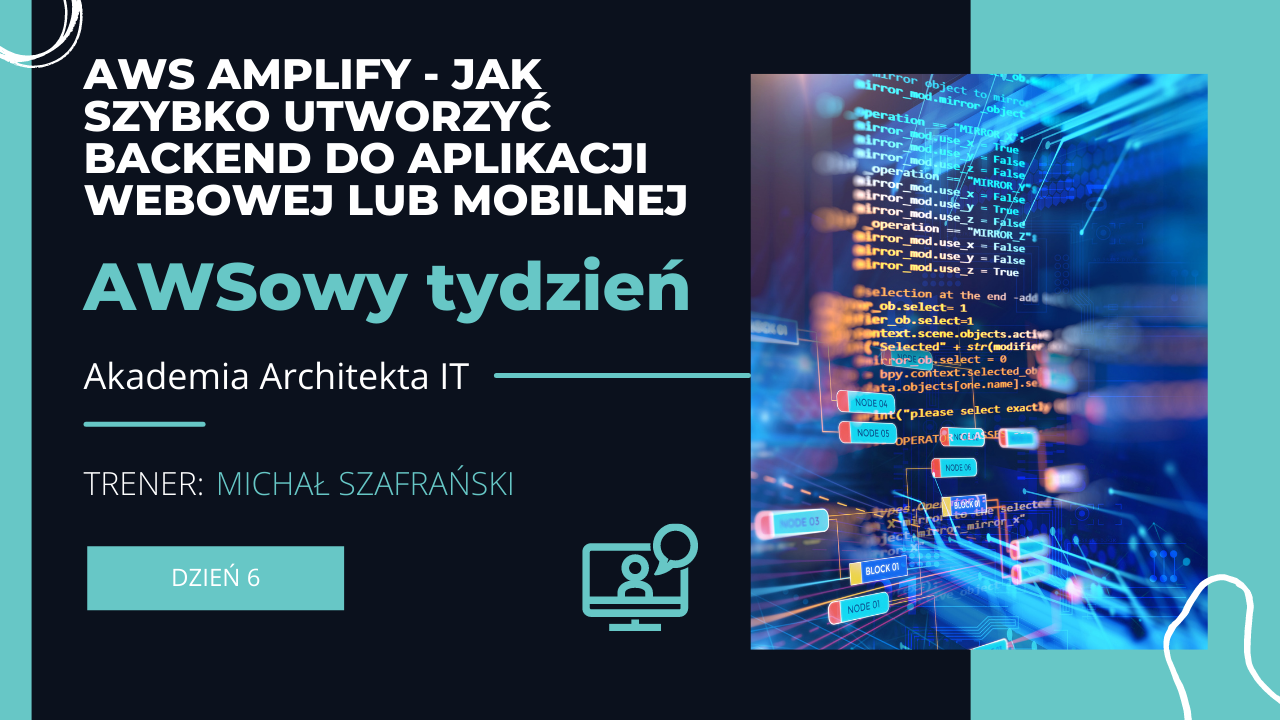
AWS Amplify – jak szybko utworzyć backend do aplikacji webowej lub mobilnej
Maży ci się zostanie milionerem za młodu? Chcesz wydać apkę, którą pokochają miliony. Z usługą AWS Amlify to łatwiejsze niż myślisz. Wystarczy, że oprogramujesz frontend, a resztę powierzysz usłudze Amplify, która zajmie się uwierzytelnieniem użytkowników, przechowywaniem danych i plików, wdrożeniami czy notyfikacjami. Na warsztatach zapoznasz się z możliwościami, jakie daje ci Amplify i zaprogramujesz własną aplikację internetową.
Warsztaty live w ramach AWSowego tygodnia ➡️ https://www.akademiaarchitektait.pl/plan-wydarzen-awsowego-tygodnia/
Wykorzystywane oprogramowanie w warsztacie
Angular
npm install -g @angular/cli
Amplify CLI
npm install -g @aws-amplify/cli
Po instalacji należy wykonać konfigurację:
amplify configure
Aplikacja Angular art-store
ng new art-store cd art-store code . ng serve --open
Start Amplify
npm install --save aws-amplify @aws-amplify/ui-angular
amplify init
Pierwsze API
amplify add api
Plik schema.graphql
type Art @model { id: ID! title: String! description: String! author: String! }
amplify push
amplify status
mv src/aws-exports.js src/aws-exports.ts
Plik main.ts
import Amplify from "aws-amplify"; import aws_exports from "./aws-exports"; Amplify.configure(aws_exports);
Plik tsconfig.app.json
"compilerOptions": { "types" : ["node"] }
Plik tsconfig.json
"strict": false,
Plik polyfills.ts
(window as any).global = window; (window as any).process = { env: { DEBUG: undefined }, };
Nowy plik src/index.d.ts
declare module 'graphql/language/ast' { export type DocumentNode = any } declare module 'graphql/error/GraphQLError' { export type GraphQLError = any }
Dodawanie obrazów
Nowy plik src/models/art.ts
export type Art = { id : string, title : string, description : string, author: string };
Plik app.components.ts
import { Component } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; import { APIService } from './API.service'; import { Art } from '../models/art'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.scss'] }) export class AppComponent { title = 'art-store'; public createForm: FormGroup; constructor(private api: APIService, private fb: FormBuilder) { } async ngOnInit() { this.createForm = this.fb.group({ 'title': ['', Validators.required], 'description': ['', Validators.required], 'author': ['', Validators.required] }); } public onCreate(art: Art) { this.api.CreateArt(art).then(event => { console.log('wpis o obrazie utworzony!'); this.createForm.reset(); }) .catch(e => { console.log('error creating art...', e); }); } }
Amplify Angular UI Module do src/app/app.module.ts
import { AmplifyUIAngularModule } from '@aws-amplify/ui-angular'; import { FormsModule, ReactiveFormsModule } from '@angular/forms'; .... imports: [ BrowserModule, AppRoutingModule, AmplifyUIAngularModule, FormsModule, ReactiveFormsModule, ],
Plik app.component.html
<div class="form-body"> <form autocomplete="off" [formGroup]="createForm" (ngSubmit)="onCreate(createForm.value)" > <div> <label>Tytuł: </label> <input type="text" formControlName="title" autocomplete="off" /> </div> <div> <label>Opis: </label> <input type="text" formControlName="description" autocomplete="off" /> </div> <div> <label>Autor: </label> <input type="text" formControlName="author" autocomplete="off" /> </div> <button type="submit">Zapisz</button> </form> </div>
ng serve --open
amplify console api
Lista obrazów
app.component.ts
arts: Array<Art>; ... this.api.ListArts().then(event => { this.arts = event.items; }); this.api.OnCreateArtListener.subscribe((event: any) => { const newArt = event.value.data.OnCreateArt; this.arts = [newArt, ...this.arts]; });
app.component.html
<hr /> <div *ngFor="let art of arts"> <h2>{{ art.title }}</h2> <h3>{{ art.author }}</h3> <h4>{{ art.description }}</h4> </div>
Uwierzytelnianie użytkowników
amplify add auth
<amplify-authenticator> <div> Sklep z antykami <amplify-sign-out></amplify-sign-out> </div> ..... </amplify-authenticator>
amplify push
amplify console
ng serve --open
Wdrożenie aplikacji
amplify add hosting amplify publish amplify console